Dev C++ Declare String
- C++ Basics
Declaring String Or Character Array in C String data type is not supported in C Programming. String means Collection of Characters to form particular word. String is useful whenever we accept name of the person, Address of the person, some descriptive information. We cannot declare string using String Data Type, instead of we use array. A string is represented by class System.String. The “string” keyword is an alias for System.String class and instead of writting System.String one can use String which is a shorthand for System.String class. So we can say string and String both can be used as an alias of System.String class. So string is an object of System.String class. @Mike: You're welcome. Please don't take this as offensive, but it looks like you're a little confused between C and C. They're actually different languages, even though one is derived largely from the other and they share a lot of the same syntax. Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value. To declare an array, define the variable type, specify the name of the array followed by square brackets and specify the number of elements it should store.
- C++ Object Oriented
The same could also be done for the scores of the other members of the class (elements of the array from index 0 to index 29). If we then wanted to use these values later (for example if we wanted to cout one or all of the elements), we can access a certain element of the array just as we did when we were assigning values to each element - by writing the array name and then the index number in. You are declaring a pointer that points to a string stored some where in your program (modifying this string is undefined behavior) according to the C programming language 2 ed. Char p2 = 'String'; You are declaring an array of char initialized with the string 'String' leaving to the compiler the job to count the size of the array. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, PHP, Python, Bootstrap, Java and XML.
- C++ Advanced
- C++ Useful Resources
- Selected Reading
C++ provides following two types of string representations −
- The C-style character string.
- The string class type introduced with Standard C++.
The C-Style Character String
The C-style character string originated within the C language and continues to be supported within C++. This string is actually a one-dimensional array of characters which is terminated by a null character '0'. Thus a null-terminated string contains the characters that comprise the string followed by a null.
The following declaration and initialization create a string consisting of the word 'Hello'. To hold the null character at the end of the array, the size of the character array containing the string is one more than the number of characters in the word 'Hello.'
If you follow the rule of array initialization, then you can write the above statement as follows −
Following is the memory presentation of above defined string in C/C++ −
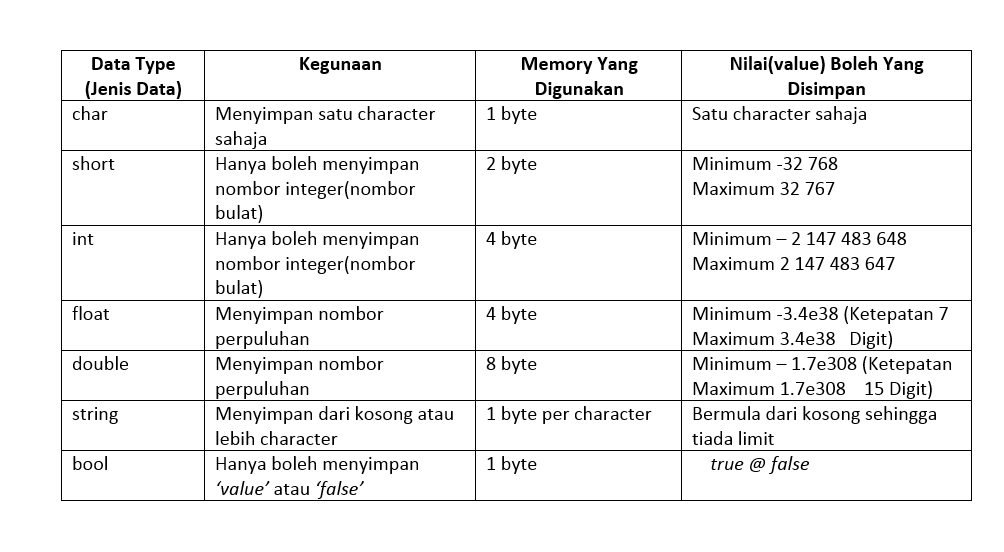
Actually, you do not place the null character at the end of a string constant. The C++ compiler automatically places the '0' at the end of the string when it initializes the array. Let us try to print above-mentioned string −
When the above code is compiled and executed, it produces the following result −
C++ supports a wide range of functions that manipulate null-terminated strings −
Sr.No | Function & Purpose |
---|---|
1 | strcpy(s1, s2); Copies string s2 into string s1. In Summary, Valhalla Full Bundle will allow yourself improve the amount of your tunes clip and far too will permit on your own difference fade in just/out outcomes. The program is involved with all 8 main vowel articulation these types of as Ah, Ee, Eh, Ih, Mm, Oh, and Oo.Trillian is the successor in direction of Spectrasonics’s Trilogy bass device and it specials further take care of of the musical time period. Cracked vst bundle. Valhalla Full Bundle Free Download Full Version LatestIt offers your self with the aid guide as properly as distinctive tutorials within just buy toward order aspects started off. |
2 | strcat(s1, s2); Concatenates string s2 onto the end of string s1. |
3 | strlen(s1); Returns the length of string s1. |
4 | strcmp(s1, s2); Returns 0 if s1 and s2 are the same; less than 0 if s1<s2; greater than 0 if s1>s2. |
5 | strchr(s1, ch); Returns a pointer to the first occurrence of character ch in string s1. |
6 | strstr(s1, s2); Returns a pointer to the first occurrence of string s2 in string s1. |
Following example makes use of few of the above-mentioned functions −
When the above code is compiled and executed, it produces result something as follows −
The String Class in C++
The standard C++ library provides a string class type that supports all the operations mentioned above, additionally much more functionality. Let us check the following example −
When the above code is compiled and executed, it produces result something as follows −
- C Programming Tutorial
- C Programming useful Resources
- Selected Reading
Arrays allow to define type of variables that can hold several data items of the same kind. Similarly structure is another user defined data type available in C that allows to combine data items of different kinds.
Structures are used to represent a record. Suppose you want to keep track of your books in a library. You might want to track the following attributes about each book −
- Title
- Author
- Subject
- Book ID
Defining a Structure
To define a structure, you must use the struct statement. The struct statement defines a new data type, with more than one member. The format of the struct statement is as follows −
The structure tag is optional and each member definition is a normal variable definition, such as int i; or float f; or any other valid variable definition. At the end of the structure's definition, before the final semicolon, you can specify one or more structure variables but it is optional. Here is the way you would declare the Book structure −
Accessing Structure Members
To access any member of a structure, we use the member access operator (.). The member access operator is coded as a period between the structure variable name and the structure member that we wish to access. You would use the keyword struct to define variables of structure type. The following example shows how to use a structure in a program −
When the above code is compiled and executed, it produces the following result −
Structures as Function Arguments
You can pass a structure as a function argument in the same way as you pass any other variable or pointer.
When the above code is compiled and executed, it produces the following result −
Pointers to Structures
You can define pointers to structures in the same way as you define pointer to any other variable −
Now, you can store the address of a structure variable in the above defined pointer variable. To find the address of a structure variable, place the '&'; operator before the structure's name as follows −
To access the members of a structure using a pointer to that structure, you must use the → operator as follows −
Let us re-write the above example using structure pointer.
When the above code is compiled and executed, it produces the following result −
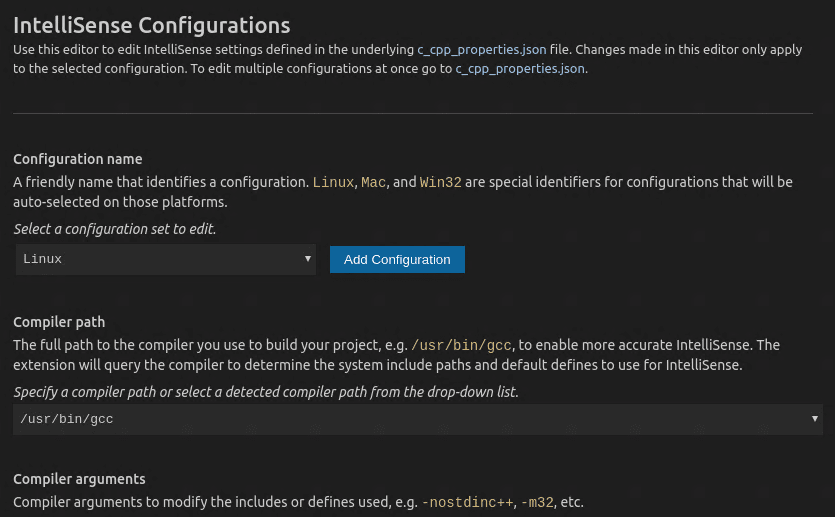
Bit Fields
C++ Declare Array Of Objects
Bit Fields allow the packing of data in a structure. This is especially useful when memory or data storage is at a premium. Typical examples include −
Packing several objects into a machine word. e.g. 1 bit flags can be compacted.
Reading external file formats -- non-standard file formats could be read in, e.g., 9-bit integers.
C allows us to do this in a structure definition by putting :bit length after the variable. For example −
Dev C Declare String Guitar
Here, the packed_struct contains 6 members: Four 1 bit flags f1.f3, a 4-bit type and a 9-bit my_int.
C++ Declare String Variable
C automatically packs the above bit fields as compactly as possible, provided that the maximum length of the field is less than or equal to the integer word length of the computer. If this is not the case, then some compilers may allow memory overlap for the fields while others would store the next field in the next word.